Solving Matrix
Equation by LatoolNet (Console Application with C#)
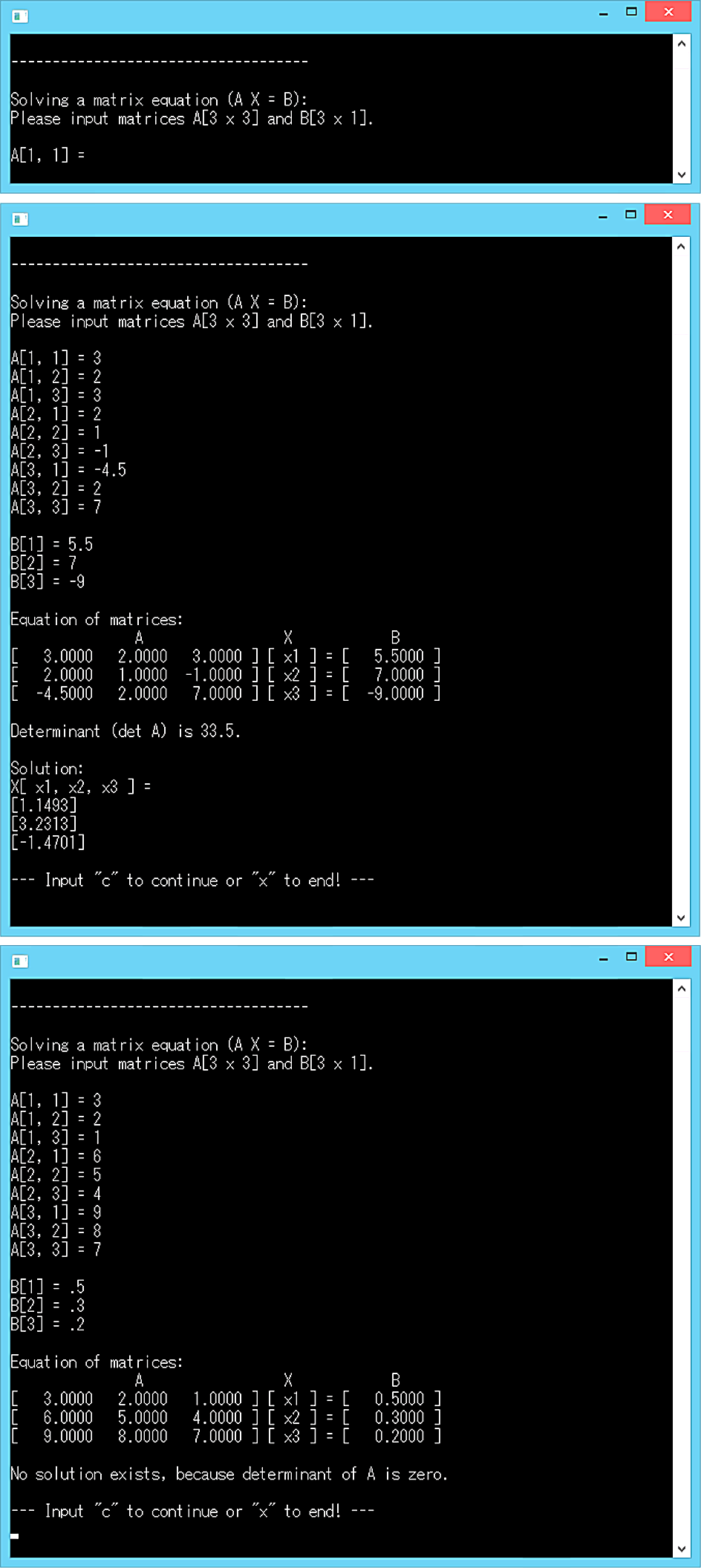
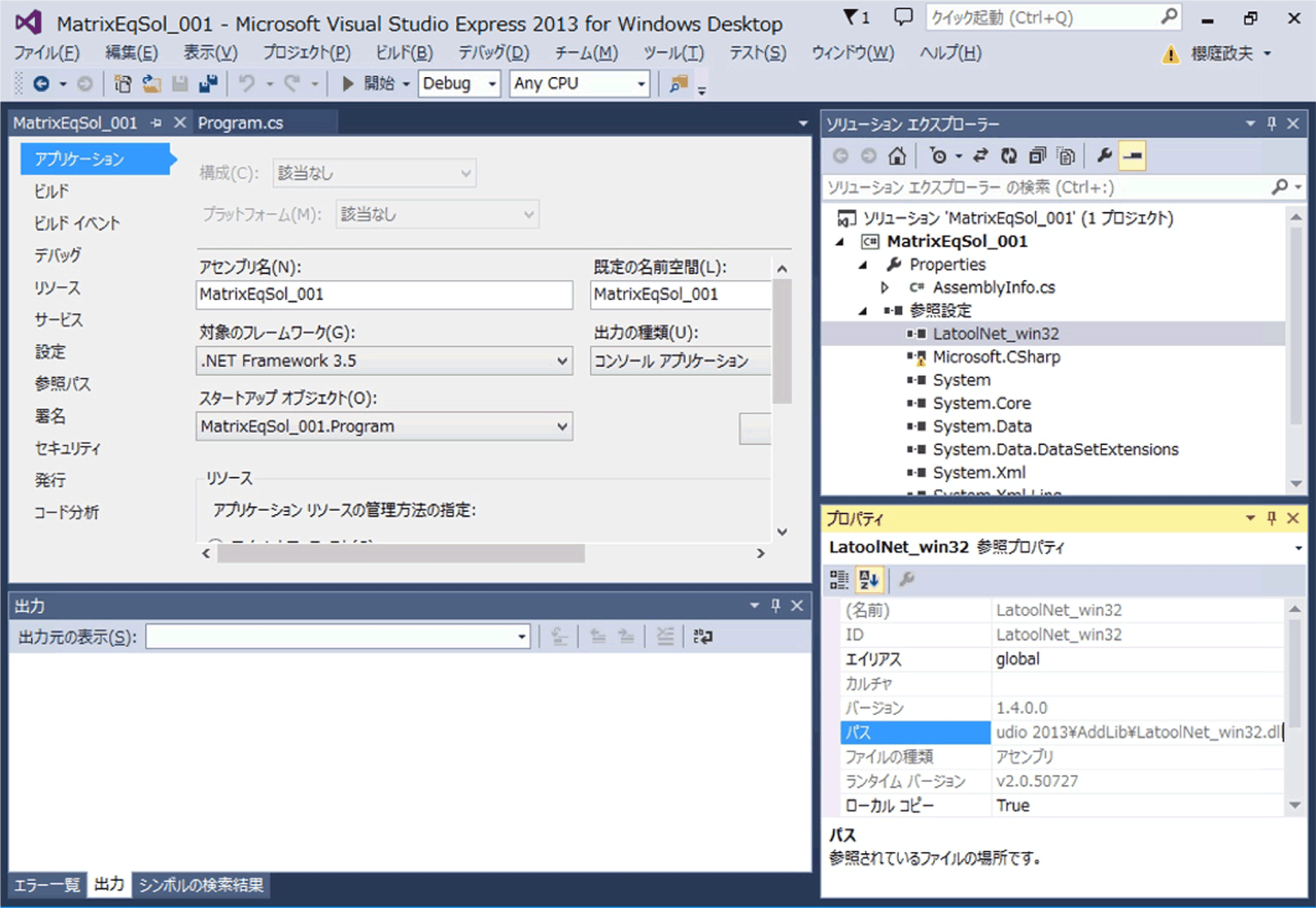
- In [Properties] in [Solution Explorer], "Taget framework" should be changed to ".NET Framework 3.5". [5,6]
//
MatrixEqSol_001\Program.cs // Solving Matrix Equation by LatoolNet (Console Application with C#) // on Microsoft Visual Studio Express 2013 for Windows Desktop // Nov. 2, 2014, by Masao Sakuraba // * NOTE1: Blue-color parts were automatically generated by Visual Studio. // * NOTE2: Red-color parts were newly added. using System; using System.Collections.Generic; using System.Linq; using System.Text; // using System.Threading.Tasks; // Unavailable beyond .NET Framework 3.5 using LatoolNet; // Only available with .NET Framework 3.5 namespace MatrixEqSol_001 { class Program { static void Main(string[] args) { START: Matrix A = new Matrix(3, 3); Matrix B = new Matrix(3, 1); Console.WriteLine("\n------------------------------------\n"); Console.WriteLine("Solving a matrix equation (A X = B):"); Console.WriteLine("Please input matrices A[3 x 3] and B[3 x 1].\n"); string a_temp; for (int j = 0; j < 3; j++) { for (int i = 0; i < 3; i++) { Console.Write("A[" + (j + 1) + ", " + (i + 1) + "] = "); a_temp = Console.ReadLine(); A[j, i] = double.Parse(a_temp); } } Console.WriteLine(); for (int k = 0; k < 3; k++) { Console.Write("B[" + (k + 1) + "] = "); a_temp = Console.ReadLine(); B[k, 0] = double.Parse(a_temp); } Console.WriteLine(); Console.WriteLine("Equation of matrices:"); Console.WriteLine(" A X B"); for (int m = 0; m < 3; m++) { Console.Write("[ " + string.Format("{0,8:f4}", A[m, 0]) + " "); Console.Write(string.Format("{0,8:f4}", A[m, 1]) + " "); Console.Write(string.Format("{0,8:f4}", A[m, 2]) + " ] [ x" + (m + 1) + " ] "); Console.Write("= [ " + string.Format("{0,8:f4}", B[m, 0]) + " ]\n"); } Console.WriteLine(); // Calculation of a determinant of matrix A. double det = 0.0; det = A[0, 0] * A[1, 1] * A[2, 2]; det += A[1, 0] * A[2, 1] * A[0, 2]; det += A[2, 0] * A[0, 1] * A[1, 2]; det -= A[2, 0] * A[1, 1] * A[0, 2]; det -= A[1, 0] * A[0, 1] * A[2, 2]; det -= A[0, 0] * A[2, 1] * A[1, 2]; if (det == 0.0) { Console.WriteLine("No solution exists, because determinant of A is zero.\n"); goto END; } else { Console.WriteLine("Determinant (det A) is {0}.\n", det); } // For A X = B, X is solved and overwritten on B. LUFactorization.Solve(A, B); Console.WriteLine("Solution:\nX[ x1, x2, x3 ] =\n" + B.ToString()); A.Dispose(); B.Dispose(); END: Console.WriteLine("--- Input \"c\" to continue or \"x\" to end! ---"); string key = Console.ReadLine(); switch (key) { case ("c"): Console.WriteLine(); goto START; case ("x"): break; } } } } |
Reference
(1)
"LatoolNet / download (LatoolNet_1.5.0, Wed Aug 8, 2012 at 8:00 AM, by Mr. Soya Hirase)",
CodePlex - Project Hosting for Open Source Software,
http://latoolnet.codeplex.com/
(2)
"「C#で学ぶ 偏微分方程式の数値解法」のサポートページ", 平瀬創也のページ,
http://soyadotnet.appspot.com/pdebook.html
(3)
"How to: Add or Remove References By Using the Add Reference Dialog
Box", Microsoft Developer Network,
http://msdn.microsoft.com/en-us/library/vstudio/wkze6zky(v=vs.120).aspx
(4) "方法: [参照の追加]
ダイアログ ボックスを使用して参照を追加または削除する", Microsoft
Developer Network,
http://msdn.microsoft.com/ja-jp/library/vstudio/wkze6zky(v=vs.120).aspx
(5) "How to:
Target a Version of the .NET Framework", Microsoft Developer Network,
http://msdn.microsoft.com/en-us/library/bb398202.aspx
(6) "方法: .NET
Framework のバージョンをターゲットにする", Microsoft
Developer Network,
http://msdn.microsoft.com/ja-jp/library/bb398202.aspx
This home page is produced by KompoZer (free and open software).