Fractal: Symmetric Galaxy
1 (WPF
with C#)
![]() |
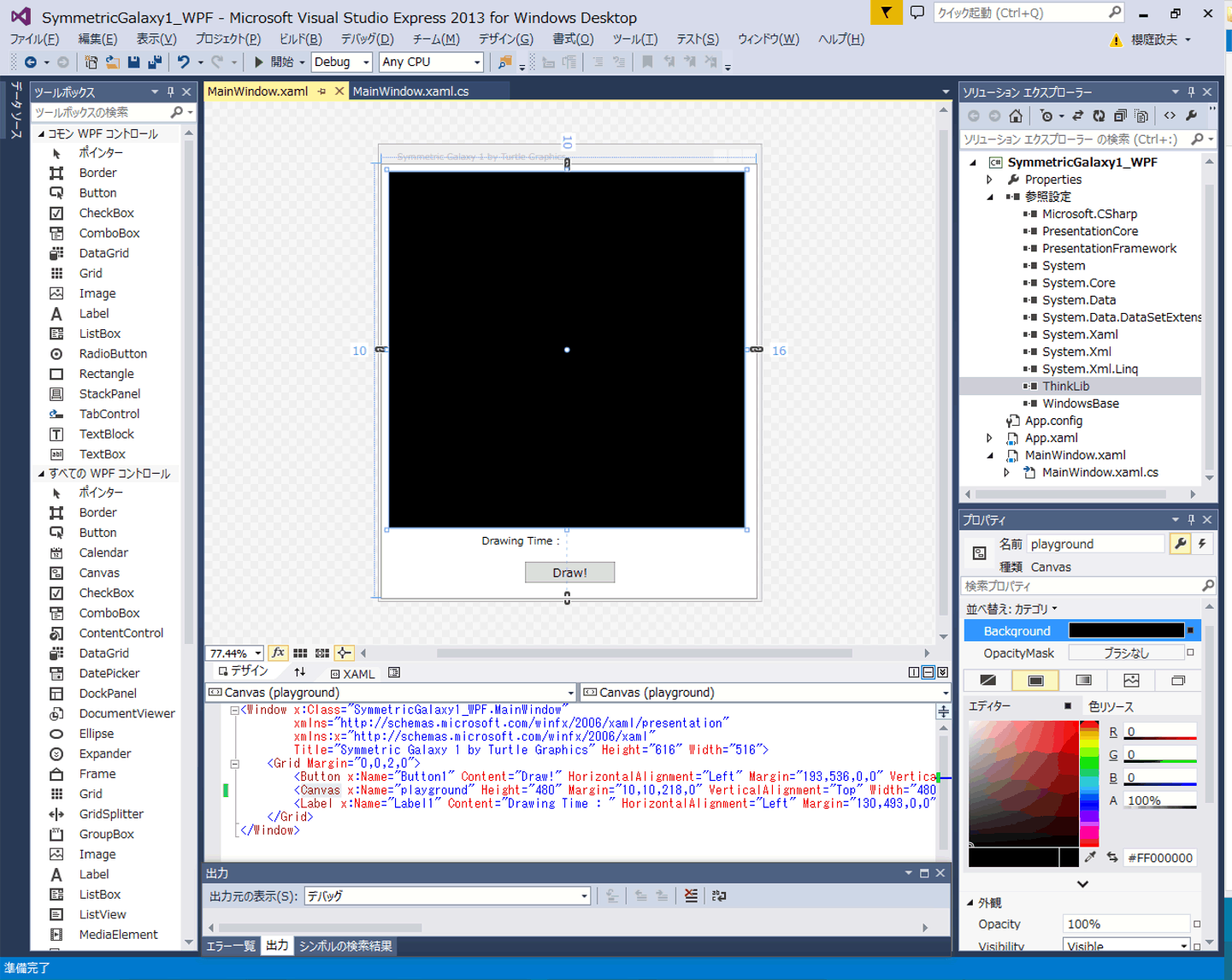
// SymmetricGalaxy1_WPF\MainWindow.xaml.cs // Symmetric Galaxy 1 with Dots // (WPF application with C# // on Microsoft Visual Studio Express 2013 for Windows Desktop) // Dec. 16, 2014, by Masao Sakuraba // (Ref. 1-6 guided me to this program.) // * NOTE1: Blue-color parts were automatically generated by Visual Studio. // * NOTE2: Red-color parts were newly added. using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Documents; using System.Windows.Input; using System.Windows.Media; using System.Windows.Media.Imaging; using System.Windows.Navigation; using System.Windows.Shapes; using System.Diagnostics; // Namespace for class "Stopwatch". using ThinkLib; // After adding the file "ThinkLib.dll" as a reference [1,2]. namespace SymmetricGalaxy1_WPF { /// <summary> /// MainWindow.xaml の相互作用ロジック /// </summary> public partial class MainWindow : Window { Turtle pen1; // Make a turtle using ThinkLib [3]. double PI = 3.141592654; double DotSize = 2.0; double Length = 220.0; double Angle = 32.0; double Scale = 1.13; int N_branch = 4; int N_repeat = 4; int Complexity = 3; void PatternGenerator1(Turtle _pen, double _length, int _complexity) { int i, j; if (_complexity == 0 | _length < 2.4) { _pen.BrushDown = false; _pen.Forward(- DotSize / 2); _pen.BrushDown = true; _pen.Forward(DotSize); _pen.BrushDown = false; _pen.Forward(- DotSize / 2); } else { for (i = 0; i < N_branch; i++) { if (i == 0) { _pen.LineBrush = Brushes.White; PatternGenerator1(_pen, _length / (2 * N_repeat + 1), _complexity - 1); } // Recursion [4,5]. if (i % N_branch == 0) _pen.LineBrush = Brushes.Yellow; if (i % N_branch == 1) _pen.LineBrush = Brushes.LightSkyBlue; if (i % N_branch == 2) _pen.LineBrush = Brushes.Fuchsia; if (i % N_branch == 3) _pen.LineBrush = Brushes.LightGreen; for (j = 1; j <= N_repeat; j++) { _pen.Forward(_length / (2 * N_repeat + 1) * Math.Pow(Scale, j - 1)); _pen.Forward(_length / (2 * N_repeat + 1) * Math.Pow(Scale, j)); _pen.Right(Angle / 2); PatternGenerator1(_pen, _length / (2 * N_repeat + 1) * Math.Pow(Scale, j), _complexity - 1); // Recursion [4,5]. _pen.Right(Angle / 2); } _pen.Right(180.0); for (j = N_repeat; j >= 1; j--) { _pen.Left(Angle); _pen.Forward(_length / (2 * N_repeat + 1) * Math.Pow(Scale, j)); _pen.Forward(_length / (2 * N_repeat + 1) * Math.Pow(Scale, j - 1)); } _pen.Right(180.0); _pen.Right(360.0 / N_branch); } } } public MainWindow() { InitializeComponent(); pen1 = new Turtle(playground); // After making Canvas in MainWindow // with the Canvas name of "playground" [3]. pen1.Visible = false; // When you do not like showning a turtle [6]. pen1.Clear(); pen1.BrushWidth = DotSize; pen1.WarpTo(240, 240); pen1.LineBrush = Brushes.Orange; pen1.Forward(DotSize); pen1.BrushDown = false; pen1.Forward(-DotSize / 2); pen1.LineBrush = Brushes.White; pen1.Left(90); } private void Button1_Click(object sender, RoutedEventArgs e) { // After making Button for Event Handler. Stopwatch TimeMeasure = new Stopwatch(); // Make a stopwatch. TimeMeasure.Start(); PatternGenerator1(pen1, Length, Complexity); double Time_in_s = (TimeMeasure.Elapsed).TotalSeconds; Label1.Content = ("Drawing Time : " + Time_in_s + " s."); } } } |
Reference
(1)
"Think Sharply with C#" by Prof. Peter Wentworth (Rhodes Univ.),
http://www.ict.ru.ac.za/resources/ThinkSharply/thinksharply/
(2) "Appendices :
Getting Started with ThinkLib",
http://www.ict.ru.ac.za/Resources/ThinkSharply/ThinkSharply/thinklib_getting_started.html
(3) "Chapter 7
: Hello, little turtles!",
http://www.ict.ru.ac.za/Resources/ThinkSharply/ThinkSharply/hello_little_turtles.html
(4) "Chapter
21 : Recursion",
http://www.ict.ru.ac.za/Resources/ThinkSharply/ThinkSharply/recursion.html
(5)
「フラクタル紀行」、芹沢浩・著、森北出版株式会社、
http://www.morikita.co.jp/books/book/107
(6)
"Appendices : ThinkLib.Turtle Documentation",
http://www.ict.ru.ac.za/Resources/ThinkSharply/ThinkSharply/thinklib_turtle_docs.html
This home page is produced by KompoZer (free and open software).